mirror of
https://git.joinsharkey.org/Sharkey/Sharkey.git
synced 2024-11-10 18:13:07 +02:00
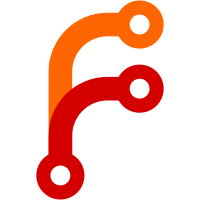
* Fix API Schema Error * Delete SimpleSchema/SimpleObj and Move schemas to dedicated files * Userのスキーマを分割してみる * define packMany type * add , * Ensure enum schema and Make "as const" put once * test? * Revert "test?" This reverts commit 97dc9bfa70851bfb7d1cf38e883f8df20fb78b79. * Revert "Fix API Schema Error" This reverts commit 21b6176d974ed8e3eb73723ad21a105c5d297323. * ✌️ * clean up * test? * wip * wip * better schema def * ✌️ * fix * add minLength property * wip * wip * wip * anyOf/oneOf/allOfに対応? ~ relation.ts * refactor! * Define MinimumSchema * wip * wip * anyOf/oneOf/allOfが動作するようにUnionSchemaTypeを修正 * anyOf/oneOf/allOfが動作するようにUnionSchemaTypeを修正 * Update packages/backend/src/misc/schema.ts Co-authored-by: Acid Chicken (硫酸鶏) <root@acid-chicken.com> * fix * array oneOfをより正確な型に * array oneOfをより正確な型に * wip * ✌️ * なんかもういろいろ * remove * very good schema * api schema * wip * refactor: awaitAllの型定義を変えてみる * fix * specify types in awaitAll * specify types in awaitAll * ✌️ * wip * ... * ✌️ * AllowDateはやめておく * 不必要なoptional: false, nullable: falseを廃止 * Packedが展開されないように * 続packed * wip * define note type * wip * UserDetailedをMeDetailedかUserDetailedNotMeかを区別できるように * wip * wip * wip specify user type of other schemas * ok * convertSchemaToOpenApiSchemaを改修 * convertSchemaToOpenApiSchemaを改修 * Fix * fix * ✌️ * wip * 分割代入ではなくallOfで定義するように Co-authored-by: Acid Chicken (硫酸鶏) <root@acid-chicken.com>
89 lines
2.6 KiB
TypeScript
89 lines
2.6 KiB
TypeScript
import { EntityRepository, Repository } from 'typeorm';
|
|
import { Page } from '@/models/entities/page';
|
|
import { Packed } from '@/misc/schema';
|
|
import { Users, DriveFiles, PageLikes } from '../index';
|
|
import { awaitAll } from '@/prelude/await-all';
|
|
import { DriveFile } from '@/models/entities/drive-file';
|
|
import { User } from '@/models/entities/user';
|
|
|
|
@EntityRepository(Page)
|
|
export class PageRepository extends Repository<Page> {
|
|
public async pack(
|
|
src: Page['id'] | Page,
|
|
me?: { id: User['id'] } | null | undefined,
|
|
): Promise<Packed<'Page'>> {
|
|
const meId = me ? me.id : null;
|
|
const page = typeof src === 'object' ? src : await this.findOneOrFail(src);
|
|
|
|
const attachedFiles: Promise<DriveFile | undefined>[] = [];
|
|
const collectFile = (xs: any[]) => {
|
|
for (const x of xs) {
|
|
if (x.type === 'image') {
|
|
attachedFiles.push(DriveFiles.findOne({
|
|
id: x.fileId,
|
|
userId: page.userId,
|
|
}));
|
|
}
|
|
if (x.children) {
|
|
collectFile(x.children);
|
|
}
|
|
}
|
|
};
|
|
collectFile(page.content);
|
|
|
|
// 後方互換性のため
|
|
let migrated = false;
|
|
const migrate = (xs: any[]) => {
|
|
for (const x of xs) {
|
|
if (x.type === 'input') {
|
|
if (x.inputType === 'text') {
|
|
x.type = 'textInput';
|
|
}
|
|
if (x.inputType === 'number') {
|
|
x.type = 'numberInput';
|
|
if (x.default) x.default = parseInt(x.default, 10);
|
|
}
|
|
migrated = true;
|
|
}
|
|
if (x.children) {
|
|
migrate(x.children);
|
|
}
|
|
}
|
|
};
|
|
migrate(page.content);
|
|
if (migrated) {
|
|
this.update(page.id, {
|
|
content: page.content,
|
|
});
|
|
}
|
|
|
|
return await awaitAll({
|
|
id: page.id,
|
|
createdAt: page.createdAt.toISOString(),
|
|
updatedAt: page.updatedAt.toISOString(),
|
|
userId: page.userId,
|
|
user: Users.pack(page.user || page.userId, me), // { detail: true } すると無限ループするので注意
|
|
content: page.content,
|
|
variables: page.variables,
|
|
title: page.title,
|
|
name: page.name,
|
|
summary: page.summary,
|
|
hideTitleWhenPinned: page.hideTitleWhenPinned,
|
|
alignCenter: page.alignCenter,
|
|
font: page.font,
|
|
script: page.script,
|
|
eyeCatchingImageId: page.eyeCatchingImageId,
|
|
eyeCatchingImage: page.eyeCatchingImageId ? await DriveFiles.pack(page.eyeCatchingImageId) : null,
|
|
attachedFiles: DriveFiles.packMany(await Promise.all(attachedFiles)),
|
|
likedCount: page.likedCount,
|
|
isLiked: meId ? await PageLikes.findOne({ pageId: page.id, userId: meId }).then(x => x != null) : undefined,
|
|
});
|
|
}
|
|
|
|
public packMany(
|
|
pages: Page[],
|
|
me?: { id: User['id'] } | null | undefined,
|
|
) {
|
|
return Promise.all(pages.map(x => this.pack(x, me)));
|
|
}
|
|
}
|