mirror of
https://git.joinsharkey.org/Sharkey/Sharkey.git
synced 2024-11-14 19:43:09 +02:00
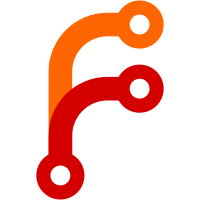
* cleanup: trim trailing whitespace * update(`.editorconfig`) --------- Co-authored-by: syuilo <Syuilotan@yahoo.co.jp>
79 lines
2.1 KiB
TypeScript
79 lines
2.1 KiB
TypeScript
import { Inject, Injectable } from '@nestjs/common';
|
|
import type { UserProfilesRepository, UsersRepository } from '@/models/index.js';
|
|
import { Endpoint } from '@/server/api/endpoint-base.js';
|
|
import { UserEntityService } from '@/core/entities/UserEntityService.js';
|
|
import { DI } from '@/di-symbols.js';
|
|
import { ApiError } from '../error.js';
|
|
|
|
export const meta = {
|
|
tags: ['account'],
|
|
|
|
requireCredential: true,
|
|
|
|
res: {
|
|
type: 'object',
|
|
optional: false, nullable: false,
|
|
ref: 'MeDetailed',
|
|
},
|
|
|
|
errors: {
|
|
userIsDeleted: {
|
|
message: 'User is deleted.',
|
|
code: 'USER_IS_DELETED',
|
|
id: 'e5b3b9f0-2b8f-4b9f-9c1f-8c5c1b2e1b1a',
|
|
kind: 'permission',
|
|
},
|
|
}
|
|
} as const;
|
|
|
|
export const paramDef = {
|
|
type: 'object',
|
|
properties: {},
|
|
required: [],
|
|
} as const;
|
|
|
|
// eslint-disable-next-line import/no-default-export
|
|
@Injectable()
|
|
export default class extends Endpoint<typeof meta, typeof paramDef> {
|
|
constructor(
|
|
@Inject(DI.usersRepository)
|
|
private usersRepository: UsersRepository,
|
|
|
|
@Inject(DI.userProfilesRepository)
|
|
private userProfilesRepository: UserProfilesRepository,
|
|
|
|
private userEntityService: UserEntityService,
|
|
) {
|
|
super(meta, paramDef, async (ps, user, token) => {
|
|
const isSecure = token == null;
|
|
|
|
const now = new Date();
|
|
const today = `${now.getFullYear()}/${now.getMonth() + 1}/${now.getDate()}`;
|
|
|
|
// 渡ってきている user はキャッシュされていて古い可能性があるので改めて取得
|
|
const userProfile = await this.userProfilesRepository.findOne({
|
|
where: {
|
|
userId: user.id,
|
|
},
|
|
relations: ['user'],
|
|
});
|
|
|
|
if (userProfile == null) {
|
|
throw new ApiError(meta.errors.userIsDeleted);
|
|
}
|
|
|
|
if (!userProfile.loggedInDates.includes(today)) {
|
|
this.userProfilesRepository.update({ userId: user.id }, {
|
|
loggedInDates: [...userProfile.loggedInDates, today],
|
|
});
|
|
userProfile.loggedInDates = [...userProfile.loggedInDates, today];
|
|
}
|
|
|
|
return await this.userEntityService.pack<true, true>(userProfile.user!, userProfile.user!, {
|
|
detail: true,
|
|
includeSecrets: isSecure,
|
|
userProfile,
|
|
});
|
|
});
|
|
}
|
|
}
|